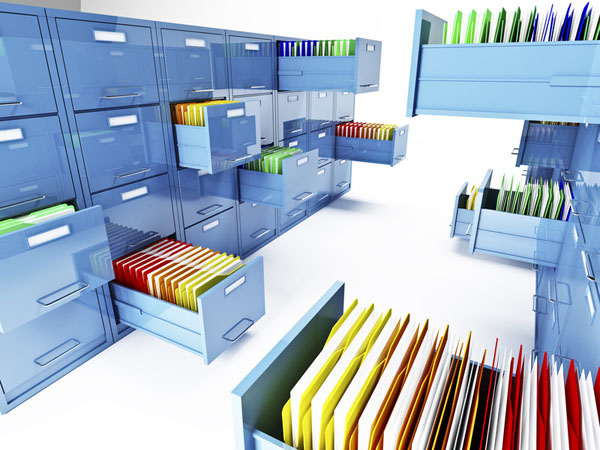
A while back I started writing about HTML5 and wrote “a brief guide to the enhancements and new features of HTML5” as well as more detailed articles about “HTML5 Video“, drawing using the “HTML5 Canvas” and “HTML5 Canvas Animation” and also one about scaleable vector graphics (SVG) with examples using HTML5. This follows on from those subjects with an article dedicated to the “HTML5 Web Storage API”.
There are two new types of web storage that are now available to web developers in HTML5 for both local and session storage. However, before I can cover the Web Storage API, I think it’s best to cover what there was before HTML5 when all the web developer really had for client side storage was cookies.
Using Cookies
A cookie is a small file that stays on your computer either temporarily or permanently. There are some limitations to what you can do with cookies but I won’t go into that here. I will also not be explaining the syntax around cookies or how they work. I have included an example below and the JavaScript in the head section of this example was taken from an online tutorial on JavaScript cookies (link).
This code will check to see if you have previously visited the page by looking for a cookie on your computer and if so it will read that cookie and welcome you back.
If you have not previously visited the page it will ask for your name and store it in a cookie for future use.
<!DOCTYPE HTML>
<html>
<head>
<meta charset=”utf-8″>
<title>Cookie Example</title>
<script type=”text/javascript”>
function setCookie(cname, cvalue, exdays) {
var d = new Date();
d.setTime(d.getTime() + (exdays*24*60*60*1000));
var expires = “expires=”+d.toUTCString();
document.cookie = cname + “=” + cvalue + “; ” + expires;
}
function getCookie(cname) {
var name = cname + “=”;
var ca = document.cookie.split(‘;’);
for(var i=0; i<ca.length; i++) {
var c = ca[i];
while (c.charAt(0)==’ ‘) c = c.substring(1);
if (c.indexOf(name) != -1) return c.substring(name.length, c.length);
}
return “”;
}
function checkCookie() {
var user = getCookie(“username”);
if (user != “”) {
alert(“Welcome again ” + user);
} else {
user = prompt(“Please enter your name:”, “”);
if (user != “” && user != null) {
setCookie(“username”, user, 365);
}
}
}
</script>
</head>
<body onLoad=”checkCookie();”>
<h1 id=”namebox”></h1>
<script type=”text/javascript”>document.getElementById(“namebox”).innerHTML = getCookie(“username”);</script>
<noscript>You need to turn on cookies for this to work</noscript>
</body>
</html>
The Web Storage API
Cookies are very popular to store two types of data; session data which stores values shared with a server and local data (as in the example above) which are used by a web page to store explicit values for a set duration on your computer. Cookies stay on the computer until you delete them but each will expire and therefore become unusable at some point in the future. Local cookies have an expiry set explicitly (like expires after 1 year) and sessions cookies expire when the link to the server is severed (usually when you close the browser or logout). Cookies are text files so they can be manipulated using a text editor.
Anyway, this article is not about cookies, it is about the new Web Storage API. As you might have guessed there are two types of web storage to cover the two cookie scenarios mentioned above; local storage and session storage.
An example using Local Storage
The methods for local storage and session storage are the same but use separate objects. Just like cookies, they are not meant to be used to store sensitive data. However, unlike cookies, they are not transmitted to the server with each web request as they are only called upon as required.
Session storage persists whilst the browser session is open after that it is removed from your computer. Local storage, on the other hand, remains on your computer for future browser sessions. Storage is set and recalled using key and value pairs; you set a key say “username” and give it a value such as “Bob” and then in code anytime you reference the storage of key “username” and it returns “Bob”.
The methods available are as follows:
clear() | This wipes out all storage for that domain |
getItem(key) | This retrieves the value for the key specified |
key(index) | This can be used to get a value based on its position in storage – it is zero based so the first item stored is index 0 and the second item is index 1 (it can be used to iterate through all items in storage when used with length) for (var i = 0; i < localStorage.length; i++) |
length | This is used to identify how many items are in storage |
removeItem(key) | This removes a particular key and value pairing from storage |
setItem(key, value) | This adds or overwrites a particular key and value pairing |
Here is an example of using local storage to set, retrieve and remove an item from local storage. It takes a user name in the input box, you click Store to save it to local storage (which also empties the input box) and click Fetch to retrieve it, which adds it to the input box so you can modify and store again if you want to. The Wipe button removes the name from storage.
Here’s the code listing for this example and as you will see it is a lot cleaner than using cookies.
<!DOCTYPE HTML>
<html>
<head>
<meta charset=”utf-8″>
<title>Web Storage API</title>
<script>
function storeName()
{
var storedname = document.getElementById(“namebox”).value;
if(storedname == “null” || storedname == “”) return false;
localStorage.setItem(“storedname”, storedname);
document.getElementById(“namebox”).value = “”;
document.getElementById(“message”).innerHTML = “‘” + localStorage.getItem(“storedname”) + “‘ is stored.”;
}
function fetchName()
{
if(localStorage.getItem(“storedname”) == null) return false;
document.getElementById(“namebox”).value = localStorage.getItem(“storedname”);
document.getElementById(“message”).innerHTML = “Name fetched is ‘” + localStorage.getItem(“storedname”) + “‘.”;
}
function wipeName()
{
if(localStorage.getItem(“storedname”) == null) return false;
var storedname = localStorage.getItem(“storedname”);
localStorage.removeItem(“storedname”);
document.getElementById(“namebox”).value = “”;
document.getElementById(“message”).innerHTML = “‘” + storedname + “‘ has been wiped.”;
}
</script>
</head>
<body>
<h1>Enter name:</h1>
<input type=”text” id=”namebox” />
<input type=”button” id=”store” value=”Store” onClick=”storeName();” />
<input type=”button” id=”fetch” value=”Fetch” onClick=”fetchName();” />
<input type=”button” id=”wipe” value=”Wipe” onClick=”wipeName();” />
<p>Log:</p>
<p style=”color:red;” id=”message”></p>
</body>
</html>
An example using Session Storage
Something like user preferences on a website would use useful to put into local storage so it is remembered each time a user visits the site and something like a shopping cart items might be best placed in session storage so it is forgotten after the user has left the site. In this next example I’ve used session storage but it could just as easily been local storage.
This example uses an editable list in place of a real shopping cart. It basically has my fruit shopping list for the week as its default; apples, pears and bananas. That is my original list and I can get to that when it loads or when I click the Original Basket or Empty Basket buttons (Empty Basket removes any customised list).
I can edit the list directly and then save it using the Save Basket button. After it is saved, I can visit the Original Basket button and then fetch it back using the Recall Basket button. The Recall won’t work if I use the Empty Basket button as that deletes my saved changes.
As it uses session storage if I quit my browser and then go back into the page it should wipe my saved changes and from the page I should not be able to recall my basket.
Here’s the code for this example:
<!DOCTYPE HTML>
<html>
<head>
<meta charset=”utf-8″>
<title>Session Storage Example</title>
<script>
function initialPage()
{
sessionStorage.setItem(“originalList”, document.getElementById(“list”).innerHTML);
}
function originalList()
{
document.getElementById(“list”).innerHTML = sessionStorage.getItem(“originalList”);
}
function saveList()
{
sessionStorage.setItem(“modifiedList”, document.getElementById(“list”).innerHTML);
}
function getList()
{
document.getElementById(“list”).innerHTML = sessionStorage.getItem(“modifiedList”);
}
function removeList()
{
document.getElementById(“list”).innerHTML = sessionStorage.getItem(“originalList”);
sessionStorage.removeItem(“modifiedList”);
}
</script>
</head>
<body onLoad=”initialPage();”>
<h1>Shopping Cart</h1>
<ul contenteditable=”true” id=”list”><li>Apples</li><li>Bananas</li><li>Pears</li></ul>
<button id=”original” onClick=”originalList();”>Original Basket</button>
<button id=”save” onClick=”saveList();”>Save Basket</button>
<button id=”fetch” onClick=”getList();”>Recall Basket</button>
<button id=”remove” onClick=”removeList();”>Empty Basket</button>
</body>
</html>
Download examples
I hope you have found this useful. I have zipped up the 3 web page files so that you can download the HTML Web Storage API examples here.
Related articles
This is part of a series of articles covering the new features of HTML5. You can find an up-to-date list of those from the Development – HTML5 category.