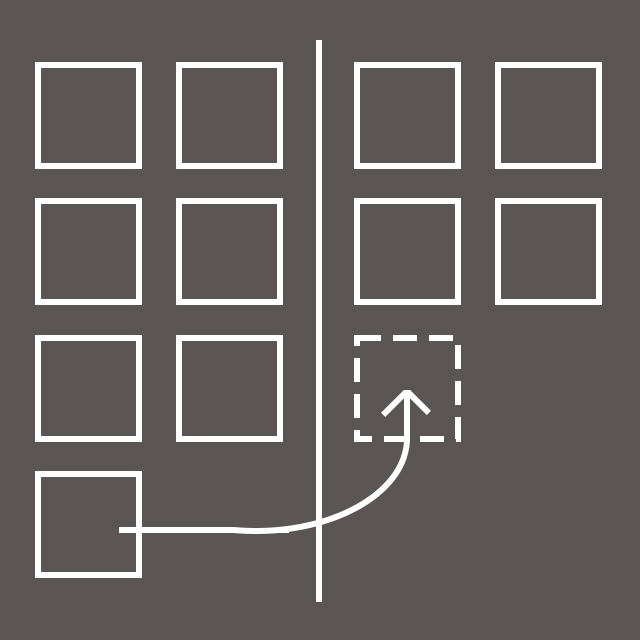
This short article walks through an example use of drag and drop on a web page using the dragNdrop API of HTML 5. Everyone should know that drag and drop means you can move an object from one place and drop it in another place on the screen so I won’t go into any more detail than that. This article is made up of a step by step guide to building an example web page to demonstrate the functionality. There are some explanations of the actions and code along the way.
The example “application” shows some images of numbers which you have to drag into an odd or even container depending whether the number is odd or even. I’ve assumed some basic HTML, CSS and JavaScript knowledge although the features used within this page are explained.
Getting started
If you want to follow along, you can download the images to use when building your page. The drag and drop example files can be downloaded here.
The zip file also has the HTML finished page but to walk along you should create your own page. You can use the images in the zip file or create your own number images yourself using a graphics editor.
To start off you will need a basic HTML 5 structure as follows.
<!doctype html>
<html>
<head>
<meta charset=”utf-8″>
<title>Drag N Drop API</title>
<style></style>
<script></script>
</head>
<body>
</body>
</html>
Make sure you include the style and script tags within the head section. We’ll add to those after the elements are added to the body section. You can use any HTML editor, even notepad. The images here are from Adobe Dreamweaver. Using dedicated HTML editing software (rather than Notepad) will usually point out error or give warnings when your entries will still run but are not HTML compliant (i.e. forgetting an alt tag on an image).
The basic steps to put together a drag and drop scenario are:
- Add the elements with an id and a class to the page using HTML.
- Add style for the page elements in the style section using CSS.
- Add the behaviours to some of the events for some elements on a page in the script section using JavaScript.
- Initialize the script when the page loads in the browser so the behaviours are added to the elements to replace their default behaviours (which is not draggable).
The page elements
First add a title and a paragraph to explain what this is all about. There are three main sections to this page and we will style them so they are next to each other. The styling will be taken care of in the next section so for now just use the class names. Each of the three sections will behave similarly but will have some colour differences. So add a dropbox class to each of the three sections (div tags). In each of these sections add another div tag and give that a class called title.
Give the first section an id of odd, a class of oddbox and within its title div enter ODD NUMBERS. Give the last section an id of even, a class of evenbox and within its title div enter EVEN NUMBERS.
Give the middle section an id of unsorted, a class of sortbox and within its title div enter TO SORT. This section also contains the number images so add those next. It is important for the simplicity of this code that the id matches the actual number represented (it just makes it easier as we want to use the value to test whether it is odd or even). Each img tag should have an alt attribute too and that could be used but it would be a little more JavaScript code. Each img tag needs to have a class of number to be properly styled.
Here is the HTML code that sits between the body tags.
<h1>Odd and even test</h1>
<p>Drag the numbers to the correct boxes.</p>
<div id=”odd” class=”dropbox oddbox”>
<div id=”test” class=”title”>ODD NUMBERS</div>
</div>
<div id=”unsorted” class=”dropbox sortbox”>
<div class=”title”>TO SORT</div>
<img id=”11″ class=”number” src=”11.png” alt=”11″>
<img id=”36″ class=”number” src=”36.png” alt=”36″>
<img id=”8″ class=”number” src=”8.png” alt=”8″>
<img id=”37″ class=”number” src=”37.png” alt=”37″>
<img id=”5″ class=”number” src=”5.png” alt=”5″>
<img id=”20″ class=”number” src=”20.png” alt=”20″>
</div>
<div id=”even” class=”dropbox evenbox”>
<div class=”title”>EVEN NUMBERS</div>
</div>
Styling the page
I’ve listed below the classes that we are using for styling the page. The important things to note are the sections float on the page to the left and any elements (apart from the title) float to the left too. The title is set to appear above each of the boxes. The sortbox has no border, whilst the text colour and border are set to red for odd and blue for even.
Here’s the CSS code that fits between the style tags.
.dropbox {
float: left;
width: 25%;
margin: 2%;
min-height: 120px;
}
.title {
margin-top: -20px;
text-align: center;
}
.number {
margin: 2px;
padding: 5px;
float: left;
border: solid thin #5a5555;
background-color: white;
}
.sortbox {
border: 0;
color: black;
}
.evenbox {
border: solid medium blue;
color: blue;
}
.oddbox {
border: solid medium red;
color: red;
}
The page events
The final piece is to add some actions to the elements on the page. I’ve created a single JavaScript function called init that is run when the page loads. That init function sets the behaviour of some of the elements on the screen. The two main ones being the odd/even sections and the number images.
First, I’ll show the code that first between the script tags and then walk through some of the important elements.
function init()
{
var numbox = document.getElementsByClassName(“number”);
var oddbox = document.getElementById(“odd”);
var evenbox = document.getElementById(“even”);
for (var i = 0; i < numbox.length; i++) {
numbox[i].ondragstart = function(event) {
event.dataTransfer.setData(“Text”, this.id);
}
}
oddbox.ondragover = function(event){ return false; }
evenbox.ondragover = function(event){ return false; }
oddbox.ondrop = function(event)
{
var num = event.dataTransfer.getData(“Text”);
var obj = document.getElementById(num);
oddbox.appendChild(obj);
if (num % 2 == 1) {
obj.style.backgroundColor = “green”;
} else {
obj.style.backgroundColor = “red”;
}
return false;
}
even.ondrop = function (event)
{
var num = event.dataTransfer.getData(“Text”);
var obj = document.getElementById(num);
evenbox.appendChild(obj);
if (num % 2 == 0) {
obj.style.backgroundColor = “green”;
} else {
obj.style.backgroundColor = “red”;
}
return false;
}
}
onload = init;
The first line within the function sets a reference in the numbox variable to each of the elements on the page that have teh class set as number (so all 6 number images). The next two lines set variables for each of the two drop sections, odd and even.
Then we have a for loop to iterate over the number images (using the numbox variable) and set an action for the ondragstart event. The action is to set some data to transfer with the object that we can use later. In this case, I’ve set some text data to be transferred with the element when dragging as the value of the elements ID. That is why it was important to give the ID of the image as the value of number shown.
Then we cancel the default ondragover functionality of the two boxes where we want to drop the number images.
Now the interesting bit is what to do for the two odd and even sections when something draggable is dropped on them. That is covered with the ondrop event. In this function I set a num variable to the value of the data transferred (i.e. the value of the number shown on the image) and also set an obj variable to represent the image being dropped.
The first action is to move the object between the parent containers. HTML takes care of this for you just by appending the element to a new parent element.
Next, I want to know if the number has been dragged into the correct box. For this I use the modulus operator %. This returns anything left over after a division. So if we divide the number by two and it has 1 left over, the number is odd and if the number left over is 0 then it is even. In the oddbox section, the background of the number image is set to green if the left over amount is 1 and red if not. In the evenbox section, 0 is green and 1 is red.
The very last thing to do after the function is to load it when the page loads. Easily accomplished with “onload = init;”.
Test it out
If all has gone well then you should be able to save your script and open the HTML page in your browser and try it out. Move a number correctly to the Odd box and then move an even number to it. See that the background changes.
Style, Script and Body code images
If anything has gone wrong, have a look at the images below. The line numbers might help you or you can have a look at the code in the download.
Next steps
Have a look at my other articles on HTML 5 here.