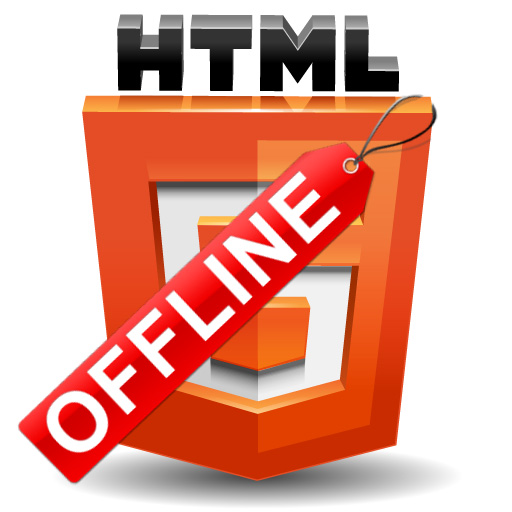
This article explains the HTML 5 “offline” feature, which gives website creators an easy method to dictate what a browser should store for use when internet (network) connectivity is unavailable. It explains the default HTTP caching method that browsers use and then how to use the HTML 5’s application cache, with a short example web application that shows an offline mode image if there is no network connection.
In this article, I’ll use “application” to mean any website, game or other type of product that is delivered via the internet to your browser. I will use “network” to mean a connection to the server that hosts the application (which would normally be the internet or local network connection for intranet applications).
Traditional offline-mode
The normal behaviour for an application rendered in a browser is that the browser connects to a web server with a request and the web server responds by returning the page to the browser to display. If the network connection is interrupted then the browser shows a network error.
Browsers actually cache a lot of the parts to a website by default, such as images and scripts and only reload these if they have changed. This is known as HTTP caching and is not new. Most browsers have an offline mode to only use HTTP caching but that just serves up what is in the local cache and might not have any content. You can see this behaviour HTTP caching behaviour if you create a website with a banner called “image1.png” and then you replace that image with another banner but keep the name the same (i.e. also “image1.png”) then the browser will show the old locally cached image when the page is reloaded. You can refresh the browser cache on Windows using Ctrl+F5. If you had the same situation where you changed the image but gave it a different filename (such as “image2.png”) then the browser would discard the locally cached version and download the new version.
Unless there is a reason that the user needs to see the latest live information, the developer of a web application can use the browser default behaviour with some JavaScript embedded in the application and have a functioning offline version.
However, utilizing HTTP caching for an offline user experience is not straight forward and relying on browser managed functionality to be compatible is risky. The authors of HTML5 chose to include an easy method to create offline applications, where you, as the application developer, take command of your user’s offline experience.
HTML5 offline web applications
If you have a site where the content does not change then you can use HTML 5 to store the pages offline. Particularly useful for say a game or web application, or a type of website that’s content doesn’t need to be the latest to fulfil its purpose. If your site frequently has new content or the user of your site is always expecting the latest information (such as live football scores) then you probably do not want to cache an offline copy. You can also have some pages and assets stored offline and others requiring a network connection. Any specified in the network section will not be rendered in the browser when offline. For those that require a network connection that are not explicitly called out when there is no connection, a fallback page or script can be actioned.
If you have an offline version then you might still need to get the latest updates or you might want to store some information until a later time when you have network connectivity so that you can send an update back to the web server. This is all possible through the HTML 5 application caching method and its cache manifest.
The cache manifest
The cache manifest contains one to three sections in a text file that has a special extension (not “.txt”). The extension you use will need to be registered on the web server. In the example at the end of this article, I use “.manifest” but you could use “.appcache”. These sections to include are CACHE (required if you want it to cache anything), NETWORK and FALLBACK (both optional). It needs to be stored in the root directory of your web application. Here is an example.
CACHE MANIFEST
# version 1.0
CACHE:
default.aspx
images/header.jpg
images/logo.jpg
styles/stylesheet.css
scripts/ajax.js
NETWORK:
scripts/adverts.js
FALLBACK:
/ error.html
The title “CACHE MANIFEST” is self-explanatory but optionally can be followed by a version number. This is important if your application will ever change in the future as the browser will check the version number it has stored against the one on the web server and if it is different it will download and use the latest version.
The three main sections are all suffixed with a colon “:”. The “CACHE:” section contains all the files to cache for offline use. You could use an asterisk “*” here but that would invalidate anything in the “NETWORK:” section, which contains the files that should not be cached. The “FALLBACK:” section contains any replacements for anything that cannot be cached. The format here is to have two file names separated by a space. In the example above, I have used the slash “/” character which has a special meaning in this section. It specifies any file that cannot be cached and, in the example above, error.html would be called in its place.
You could just have a cache and fallback section in your cache manifest file and everything that is not specified on the cache section is assumed to be an online file handled in the fallback section.
Example building an offline site
The basic steps to put together an offline application are:
- Create a cache manifest (a text file with a different file extension)
- Add page references to it
- Configure the web server to recognise the cache manifest
- Use Javascript to check network connectivity and reload if necessary
Creating the example application
I am not going to create a full-blown application here. All I will be doing is creating a very simple website to demonstrate the functionality.
The application is made up of an HTML file, a stylesheet, two images and an application cache manifest file. Two files are images but the code is shown here for all three files that can be created using any text editor.
index.html
<!doctype html>
<html lang=”en” manifest=”appcahe.manifest”>
<head>
<meta charset=”utf-8″>
<title>HTML 5 Offline Application</title>
<link href=”style.css” rel=”stylesheet” type=”text/css”>
<div class=”container”>
<div class=”header”><img src=”online.png” alt=”HTML 5 Logo” width=”180″ height=”90″ /></div>
<div class=”content”>
<h1>Welcome</h1>
<p>This page is has an offline cache mode so that is access to the server is interrupted you can still use it.</p>
</div>
</div>
</body>
</html>
style.css
@charset “utf-8”;
body {
font: 100%/1.4 Verdana, Arial, Helvetica, sans-serif;
background-color: #cacaca;
margin: 0;
padding: 0;
color: #000000;
}
a img {
border: none;
}
.container {
width: 960px;
background-color: #ffffff;
margin: 0 auto;
}
.header {
background-color: #ffffff;
}
.content {
padding: 10px 0;
}
appcahe.manifest
CACHE MANIFEST
# version 1.0
CACHE:
index.html
style.css
offline.png
FALLBACK:
online.png offline.png
These application cache example files and images can all be downloaded in a zip file here html5-offline web example files.
Enabling the Cache Manifest
You will need to make sure your server has an associated mime type. If you have access to Apache or IIS server settings then you can add a mime type for text/cache-manifest with the file extension that you have used. For example: the mime type “text/cache-manifest” might have an extension “.appcache”.
As I am putting this example on a shared hosting server where I do not have web server settings access, I will add an .htaccess file to the root directory (I have also included this htaccess file in the link to the zip file for the example website). The code in the .htaccess file is.
.htaccess
AddType text/cache-manifest manifest
Trying out the example application
So, trying it out, upload your files to a server. If you will be using Google Chrome browser then use a secure server. If you want to try it out without uploading files yourself then use this link https://www.pathowe.co.uk/other/offline/.
The steps for trying it out are as follows.
First go to the page online.
Refresh the page (F5 on Windows).
Enable your internet connection.
Refresh the page and watch the browser revert back to the online version.
The refresh side could be handled automatically with a little Javascript but that’s for another day.
Next steps
HTML5 offline cache is a very powerful and easy to use tool in your application development armoury. Obviously, if can do a lot more than switching images. Some examples might be that you tell it to only load certain content say, advertisements when online and leave whitespace when not. You can serve up a default catch-all nice explanatory error page for non-cached content in offline mode. You can allow your site to fully function offline and then save inputs back to the server when online and even do that automatically.