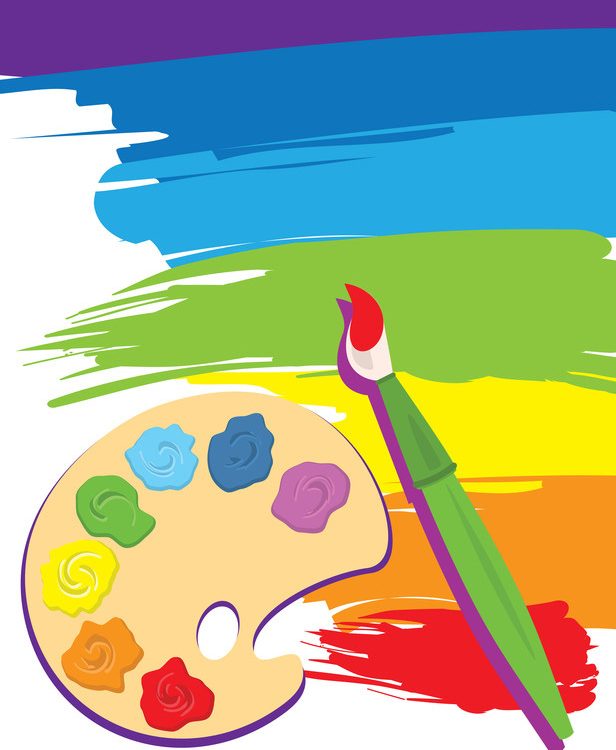
Following on from my article Getting started with HTML5 canvas, this short article adds another example of what you can do. If you haven’t already done so, it is worth revisiting my previous article to get familiar with the terms and Canvas setup.
This article shows you that animation on the HTML 5 Canvas is really quite simple. The example here moves a small box around the screen and changes its direction and colour when it hits the boundary of the canvas.
As there is not much to it so this brief article will include the full code, a couple of screenshots and explain what is happening.
HTML 5 Code
<!doctype html>
<html lang=”en”>
<head>
<meta charset=”utf-8″ />
<title>HTML 5 Canvas Animation</title>
<script>
function O(obj)
{
if (typeof obj == ‘object’)
return obj;
else
return document.getElementById(obj);
}
function S(obj)
{
return O(obj).style;
}
function animate() {
pic = O(‘canvas1’);
// canvas tag only supports width and height but you can still style the canvas
S(pic).background = ‘#cacaca’;
// canvas has no drawing abilities of its own (it is a container for graphics) so need a context
context = pic.getContext(‘2d’);
w = 590; h = 140;
x = 5; y = 5; dx = 5; dy = 5;
function move()
{
if ((x+dx > w) || (x+dx < 0)) dx = -dx;
if ((y+dy > h) || (y+dy < 0)) dy = -dy;
x += dx;
y += dy;
draw();
}
function draw()
{
if (dy < 0) {
if (dx < 0) col = ‘Red’;
else col = ‘Blue’;
} else
if (dx < 0) col = ‘Green’;
else col = ‘Yellow’;
}
context.fillStyle = col;
context.fillRect(x, y, 10, 10);
}
setInterval(move, 20);
}
</script>
</head>
<body onLoad=”animate();”>
<h1 id=”title”>HTML 5 Canvas Animation</h1>
<canvas id=”canvas1″ width=”600″ height=”150″>
This web page uses the HTML5 canvas element, which is available on most modern browsers. Please upgrade your existing browser or download a modern browser such as <a href=”https://www.google.com/intl/en_uk/chrome/browser/”>Google Chrome</a>.
</canvas>
</body>
</html>
HTML 5 Canvas Animation Explanation
The page sets up in the same way as the previous canvas examples with the usual JavaScript included for easier working with objects and styles. The canvas in this example is 600 pixels wide by 150 high and I have added the “animate” function in the body tag.
The “animate” function sets up some variables for the canvas and canvas context to work with as well as some variables for width, height positioning and direction. The “animate” function also includes its own “move” and “draw” functions. The “move” function clears the current position then checks what the new co-ordinates will be and if they exceed the canvas they sign of the direction (dx or dy) is reversed. It then makes the positioning adjustment and calls the “draw” function. The “draw” function checks to see which direction the square is travelling and adjusts its colour between yellow (down and right), blue (up and right), green (down and left) or red (up and left).
The final command of the “animate” function is setInterval(move, 20). This calls the “move” function every 20 milliseconds.
You can also download the example HTML5 Canvas animation code here.
Related articles
This is part of a series of articles covering the new features of HTML5. You can find an up-to-date list of those from the Development – HTML5 category.